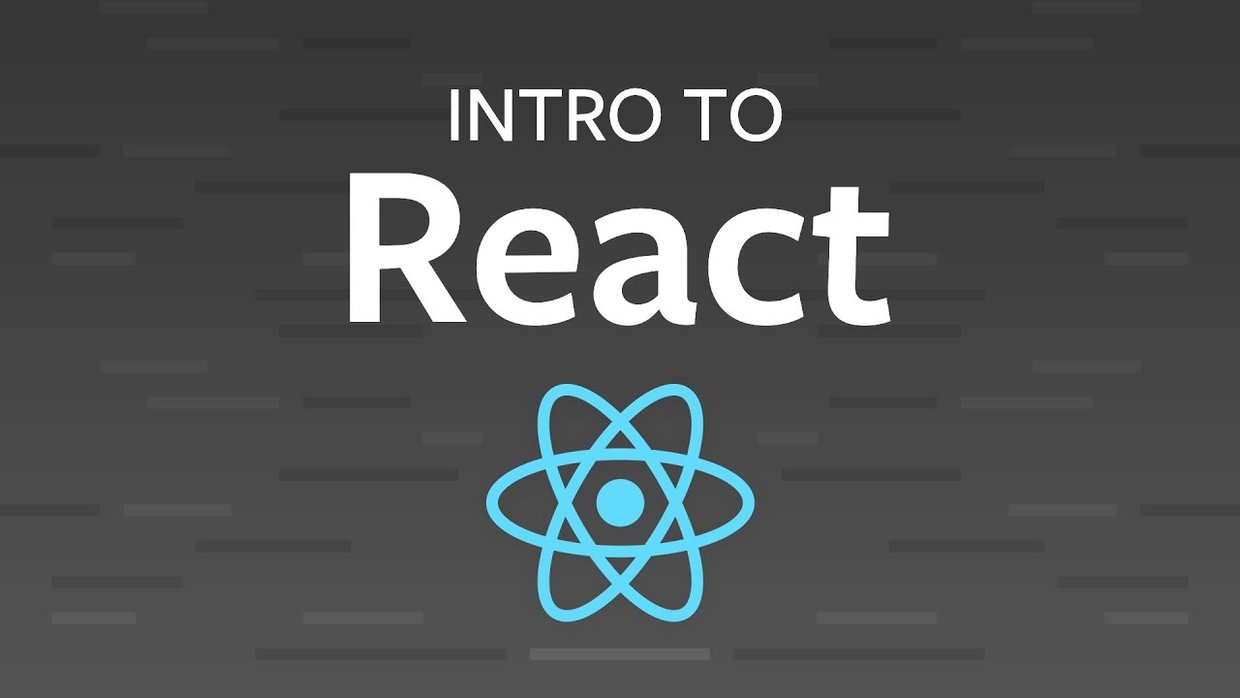
It is the most widely used library in the frontend community. Vue and Angular also exist. Once you learn one, it's not difficult to learn the others, especially React and Vue are similar.
In this article, we'll take a look at the general theory, such as what React is; why it's used; who its competitors are; what makes React fast; what are the virtual DOM structure and component structure inside React. Afterwards, we'll see the React setup. We'll touch on topics like what the project structure is.
What is React?
It is a JS library. That is, it's a library, not a framework.
The difference between Framework and Library: A framework is rigid, an ecosystem that contains everything. For example, Angular is a framework. It's like buying a closed box product that has everything inside; charger, spare parts, etc. A library, on the other hand, offers you the product but doesn't provide the accessories. Frameworks are more complex. A library offers some things, but you're not obligated to use them. It gives you more freedom, you can choose whatever you want as third-party, take it and use it. There's also a framework developed for React: NEXT.JS. It says, come use React with me, I'll provide you with an environment where everything is set up.
—
It was first released by Facebook in 2011. Initially, it wasn't well-received, but it started gaining acceptance after Facebook made it open source.
Another important feature is that it's fast due to the virtual DOM and it's component-based.
React is the library with the syntax closest to modern JS. Vue has also started to approach this. Angular has many class structures, is a bit more like TypeScript, and has its own unique structures, making it a bit more complex. These aspects are less prevalent in React.
It supports ES6 and later versions. For example, you cannot use React with ES5. In later versions, it converts the code we write to ES6 using transpilers like Babel. We use the React library to write effective, high-performance UI.
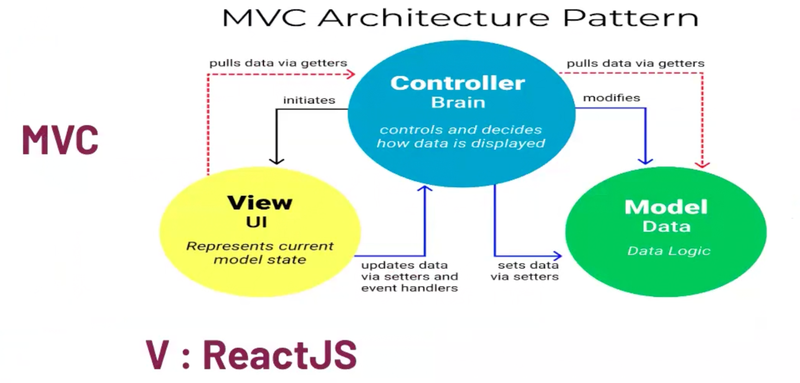
MVC: Model-View-Controller
This is a design pattern that has existed since the 1970s. MVC is a well-known architecture.
Model is the part where data resides; this concerns the backend. Controller is the API side. It establishes the connection between Model and View. Look at the incoming request, analyze it, understand what it corresponds to on the data side, and send the necessary SQL commands accordingly. The View layer can be referred to as the UI layer. React works in the View layer. It presents the data coming from the backend to the user by visualizing it. Vue and Angular also work in the View layer. Vue actually means "View" in French.
So why don't we do it with plain JS, why do we need React?
It can be done actually. React is also based on JS. But there are some structures developed by libraries like React.
- Component
These allow codes to be much shorter and more performant compared to Vanilla JS. It better applies the DRY (Don't Repeat Yourself) principle. That is, use it, don't write it over and over again. Write it once, use it in many places. A component is a building block made up of HTML-CSS-JS, like a button, etc. By writing with React, we use these structures that already exist. We don't write everything from scratch.
- Virtual DOM
It provides performance improvement.
In JS, we perform our operations through DOM Manipulation. We capture an element with commands like getElementById. We can change its style, create new elements inside it with createElement. When DOM Manipulations of applications increase, slowdown occurs. In simple applications, there isn't much data flow, but as the application grows, slowdown will occur. However, if large data and fast flow are required, DOM Manipulation causes serious slowdown. DOM is an external API running in the browser. You say "I need this part," and DOM prints it all. When you say "I need a change in another place again," it rewrites everything. Think about constantly reprinting, as a result, it slows down. We see a delay on the screen.
If you do a lot of DOM manipulation, you'll slow down a lot.
The solution here is to reduce DOM Manipulation as much as possible. And they came up with Virtual DOM.
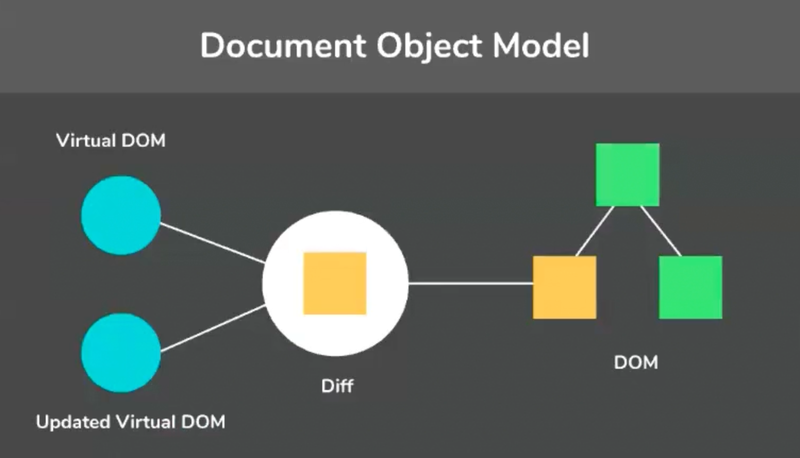
The right side is the real DOM. The left side is the client side. React says I take real copies of the real DOM. It takes 2 copies. This is called a Snapshot. You can think of it as taking a photo, so to speak. And it stores this information in the Client's RAM. It keeps it on the user's computer. The first copy is generally called the shadow DOM. The second copy is called the virtual DOM.
Normally, when a button is pressed, the resulting change goes directly to the DOM. But in React, you don't deal directly with the DOM. In React, there's actually an intermediate layer. It applies this change not to the DOM, but to the virtual DOM. In the virtual DOM, it even waits for sequential operations and writes them too. We call this Batch Updated. It waits for related events. It waits for the 1st and 2nd changes within the same event. Then it compares this virtual DOM we wrote with the shadow DOM. There's an algorithm here, we call it the Diff algorithm, this algorithm finds the difference. And in the normal DOM, it only finds and updates this detected changing part. It doesn't print the whole thing like in classic JS.
To summarize, DOM Manipulations are time-consuming operations. Especially if done one after another, React Developers said let's reduce DOM Manipulation, let's have a system that acts as a buffer, let's take copies of the DOM and throw them into the user's RAM — read and write operations in RAM are done faster, while the real DOM is like an HDD — then when there's a difference, let's detect this on the client side with the Diff algorithm and if there are going to be consecutive changes, let's wait for them too, i.e., batch updated. And let's only update the changing parts in the real DOM.
React does this Virtual DOM thing best, there are similar structures in Vue and Angular, but React is the most successful. In fact, React creates this out of necessity. Facebook is an application where there's a lot of content flow. When it slows down enough to disrupt the flow, they think about it and build such an algorithm. So it emerges out of necessity.
Also, having many components, updating very small pieces makes the virtual DOM's job easier, because a change occurs in a smaller piece, and allows it to update faster. In React, this component and virtual DOM work together to increase efficiency and speed.
Who Uses React?
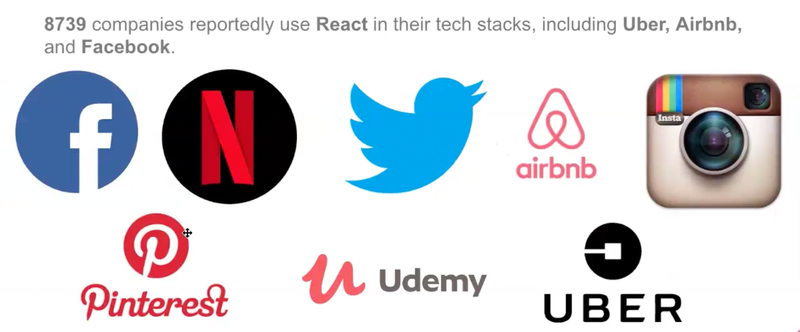
Many large companies use React, especially in areas where speed is required. In Turkey, Getir, Trendyol, and Hepsiburada were built with React. So, many big companies use React. You can see which sites use React with React Developer Tools.
Why should React be preferred?
It's easy to read and understand.
It's component-based
It allows for quick updates
Once learned, applications can be developed quickly
You can develop applications for mobile devices with React Native, which is 70% - 80% the same as React
It has a strong community.
It supports modern JS
It supports a structure called JSX (JavaScript XML). We use JS and HTML together without innerHTML and backticks.
Are there any disadvantages to React?
It may not be necessary to use React everywhere. This is actually not just a problem with React, Vue and Angular are the same. These libraries are not SEO-friendly. Browsers struggle to collect data from these applications. These applications are known as client-side applications, meaning they run on the client's computer. Therefore, they work on the client side rather than the server side. Normal classic applications generally run on the server side. So a bot goes to a single server, gets the data, pulls it, and indexes it. But in React applications, everything runs on the clients. There's little data on the server. So instead of going to one place, it goes to n number of places. This makes the bot's job quite difficult. This means you may need to do extra things for SEO in React. The reason Next.js emerged was for that SEO optimization.
React Setup
- We generally use Vite; Vite means "fast" in French. It uses Rollup as a bundler.
npm create vite@latest
*file_name
* React
* Javascript
2. Method create-react-app This is generally used for installation. Either Yarn or npm can be used.
npx create-react-app dosya-adi
yarn create-react-app dosya-adi
They all use webpack as a bundler.
3. Method: It can be initiated with Next.js
npm create-next-app@latest
STARTING AN EXISTING PROJECT
When you download a project from somewhere, it comes without node_modules, so we say npm install.
Afterwards, we run it: npm start || yarn start
npm i && npm start
we run these two commands
ADDING A NEW DEPENDENCY TO THE PROJECT (LIBRARY)
npm install axios tailwind
yarn add bootstrap react-router
pnpm add @mui
BUILD
npm run build (BUNDLE): Multiple files are combined into a single file.
TEST
npm run test: This runs the test commands. It's generally referred to as unit testing. JEST is used, and for VITE there's VITEST. Unit tests are expected to be done by developers. Other tests like E2E, Smoke, Regression, UAT (done by Product Owner, PM or BA) are expected to be done by testers.
Leave a reply